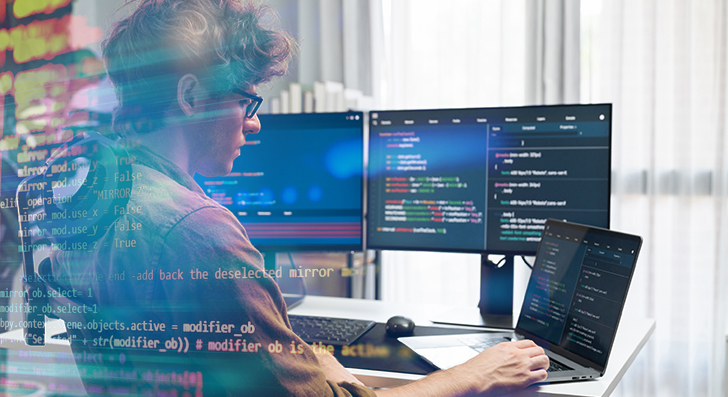
Scalability indicates your application can take care of development—more buyers, far more info, and much more visitors—without breaking. For a developer, constructing with scalability in your mind saves time and worry later on. Right here’s a transparent and useful manual to help you get started by Gustavo Woltmann.
Design for Scalability from the beginning
Scalability is not something you bolt on later on—it should be section of your respective prepare from the beginning. Numerous purposes fall short when they improve quick for the reason that the initial structure can’t manage the additional load. For a developer, you have to Assume early about how your technique will behave stressed.
Begin by coming up with your architecture to become versatile. Stay away from monolithic codebases wherever anything is tightly linked. As an alternative, use modular design or microservices. These styles crack your app into lesser, impartial pieces. Each and every module or service can scale By itself without having influencing The entire technique.
Also, contemplate your databases from day one. Will it require to take care of 1,000,000 consumers or simply 100? Select the right kind—relational or NoSQL—according to how your facts will improve. Approach for sharding, indexing, and backups early, Even when you don’t require them nevertheless.
Yet another crucial position is to stay away from hardcoding assumptions. Don’t generate code that only will work less than present problems. Take into consideration what would come about If the user foundation doubled tomorrow. Would your app crash? Would the databases decelerate?
Use style and design styles that guidance scaling, like concept queues or party-pushed units. These enable your application handle far more requests without finding overloaded.
When you Establish with scalability in mind, you're not just getting ready for achievement—you might be lowering foreseeable future problems. A properly-prepared technique is easier to maintain, adapt, and increase. It’s far better to prepare early than to rebuild later.
Use the proper Database
Deciding on the ideal databases is often a vital Element of setting up scalable purposes. Not all databases are created the identical, and using the wrong you can sluggish you down or perhaps cause failures as your application grows.
Start off by knowing your data. Can it be very structured, like rows in a desk? If Of course, a relational database like PostgreSQL or MySQL is a superb healthy. These are typically robust with relationships, transactions, and regularity. They also aid scaling tactics like read through replicas, indexing, and partitioning to handle additional site visitors and data.
When your information is a lot more flexible—like person activity logs, merchandise catalogs, or paperwork—consider a NoSQL selection like MongoDB, Cassandra, or DynamoDB. NoSQL databases are better at dealing with significant volumes of unstructured or semi-structured info and will scale horizontally much more quickly.
Also, consider your read through and compose styles. Have you been executing plenty of reads with less writes? Use caching and skim replicas. Are you currently dealing with a major create load? Check into databases that can cope with higher compose throughput, or maybe party-based info storage devices like Apache Kafka (for non permanent information streams).
It’s also sensible to Assume ahead. You may not need to have Highly developed scaling features now, but choosing a database that supports them implies you gained’t will need to modify afterwards.
Use indexing to hurry up queries. Steer clear of needless joins. Normalize or denormalize your knowledge determined by your obtain styles. And normally monitor databases performance as you develop.
In brief, the ideal databases depends on your application’s composition, velocity desires, And just how you be expecting it to improve. Acquire time to choose correctly—it’ll help you save loads of hassle afterwards.
Enhance Code and Queries
Rapidly code is vital to scalability. As your app grows, each small hold off provides up. Inadequately published code or unoptimized queries can decelerate efficiency and overload your program. That’s why it’s vital that you Develop efficient logic from the beginning.
Start off by composing thoroughly clean, easy code. Steer clear of repeating logic and take away just about anything unwanted. Don’t select the most sophisticated Answer if a straightforward one particular operates. Keep your capabilities limited, focused, and straightforward to check. Use profiling resources to find bottlenecks—destinations in which your code takes far too prolonged to run or works by using a lot of memory.
Next, check out your database queries. These often sluggish issues down much more than the code itself. Be certain Each and every question only asks for the data you truly require. Prevent Choose *, which fetches anything, and as a substitute choose distinct fields. Use indexes to hurry up lookups. And stay away from accomplishing too many joins, In particular across huge tables.
For those who discover the exact same data getting requested over and over, use caching. Retail store the outcome quickly using equipment like Redis or Memcached therefore you don’t have to repeat pricey functions.
Also, batch your databases functions when you can. As opposed to updating a row one after the other, update them in groups. This cuts down on overhead and helps make your app additional economical.
Make sure to test with huge datasets. Code and queries that operate high-quality with a hundred records may crash after they have to take care of one million.
In short, scalable apps are quickly apps. Maintain your code restricted, your queries lean, and use caching when essential. These techniques assistance your software continue to be sleek and responsive, at the same time as the load raises.
Leverage Load Balancing and Caching
As your app grows, it has to deal with a lot more consumers and a lot more targeted traffic. If anything goes as a result of a person server, it will eventually immediately turn into a bottleneck. That’s wherever load balancing and caching can be found in. Both of these applications enable keep the application quickly, stable, and scalable.
Load balancing spreads incoming visitors across various servers. In lieu of a person server executing the many operate, the load balancer routes end users to unique servers determined by availability. This implies no single server receives overloaded. If one particular server goes down, the load balancer can ship traffic to the Many others. Instruments like Nginx, HAProxy, or cloud-based mostly options from AWS and Google Cloud make this easy to build.
Caching is about storing knowledge temporarily so it can be reused immediately. When people request the same facts once again—like an item site or even a profile—you don’t need to fetch it with the database when. It is possible to serve it with the cache.
There's two typical types of caching:
one. Server-facet caching (like Redis more info or Memcached) merchants information in memory for rapid access.
two. Consumer-facet caching (like browser caching or CDN caching) merchants static files near to the person.
Caching lowers databases load, increases pace, and makes your application much more successful.
Use caching for things that don’t transform frequently. And normally ensure your cache is current when info does improve.
In a nutshell, load balancing and caching are very simple but effective resources. Jointly, they assist your app take care of extra consumers, keep fast, and Recuperate from complications. If you plan to expand, you require both.
Use Cloud and Container Equipment
To develop scalable purposes, you may need applications that let your app expand quickly. That’s where by cloud platforms and containers come in. They give you versatility, lessen set up time, and make scaling much smoother.
Cloud platforms like Amazon Net Companies (AWS), Google Cloud System (GCP), and Microsoft Azure Enable you to hire servers and solutions as you will need them. You don’t really need to invest in components or guess future capacity. When visitors raises, it is possible to incorporate far more methods with just a couple clicks or mechanically working with vehicle-scaling. When website traffic drops, you could scale down to economize.
These platforms also provide providers like managed databases, storage, load balancing, and safety resources. You are able to concentrate on developing your app in lieu of running infrastructure.
Containers are A further critical Device. A container deals your app and everything it really should operate—code, libraries, options—into 1 unit. This makes it quick to maneuver your app between environments, from a laptop computer towards the cloud, without surprises. Docker is the preferred Resource for this.
When your application works by using several containers, tools like Kubernetes assist you take care of them. Kubernetes handles deployment, scaling, and recovery. If a person part of your respective app crashes, it restarts it automatically.
Containers also help it become simple to different areas of your app into expert services. You'll be able to update or scale parts independently, that's great for effectiveness and reliability.
To put it briefly, making use of cloud and container applications signifies you can scale rapidly, deploy simply, and Get better swiftly when problems come about. If you want your app to grow with no restrictions, commence applying these resources early. They help save time, reduce chance, and assist you remain centered on setting up, not fixing.
Keep an eye on Every thing
When you don’t monitor your application, you gained’t know when matters go Incorrect. Monitoring will help the thing is how your application is carrying out, place difficulties early, and make better choices as your app grows. It’s a essential Element of building scalable methods.
Start off by monitoring essential metrics like CPU use, memory, disk Room, and reaction time. These inform you how your servers and products and services are doing. Resources like Prometheus, Grafana, Datadog, or New Relic can assist you acquire and visualize this facts.
Don’t just observe your servers—observe your application too. Keep an eye on how long it will take for customers to load webpages, how often mistakes take place, and in which they arise. Logging equipment like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly can assist you see what’s going on within your code.
Build alerts for significant challenges. One example is, If the reaction time goes previously mentioned a limit or even a support goes down, you need to get notified instantly. This assists you fix issues speedy, normally in advance of end users even observe.
Monitoring is also practical any time you make alterations. When you deploy a whole new characteristic and see a spike in faults or slowdowns, it is possible to roll it back before it results in true injury.
As your application grows, website traffic and info increase. Without the need of monitoring, you’ll miss indications of problems till it’s much too late. But with the best tools set up, you remain on top of things.
In brief, checking can help you keep your application dependable and scalable. It’s not just about recognizing failures—it’s about understanding your process and making sure it really works well, even stressed.
Final Feelings
Scalability isn’t only for huge providers. Even tiny applications require a robust foundation. By planning carefully, optimizing properly, and utilizing the correct instruments, you are able to Create applications that expand smoothly without having breaking stressed. Start modest, Imagine huge, and Make intelligent.